Coupling is a measure of how dependant two systems are on one another. Tight coupling means that i.e. most classes of the PlayerController access different Classes of the UI.
This has lots of downsides. For example if you need to change the HP-Display from a number to a HP-Bar you may have to change dozens of scripts due to a snowballing effect. By that I mean that the change in the UI-Script leads to changes in the PlayerController-Script which again lead to changes in the Enemy-Script which then lead to changes in the GameManager and the LevelManager, etc.
This is obviously very bad because the chance of adding a bug along the way grows with the number of changes we have to make. So tight coupling is something we should try to avoid as much as possible.
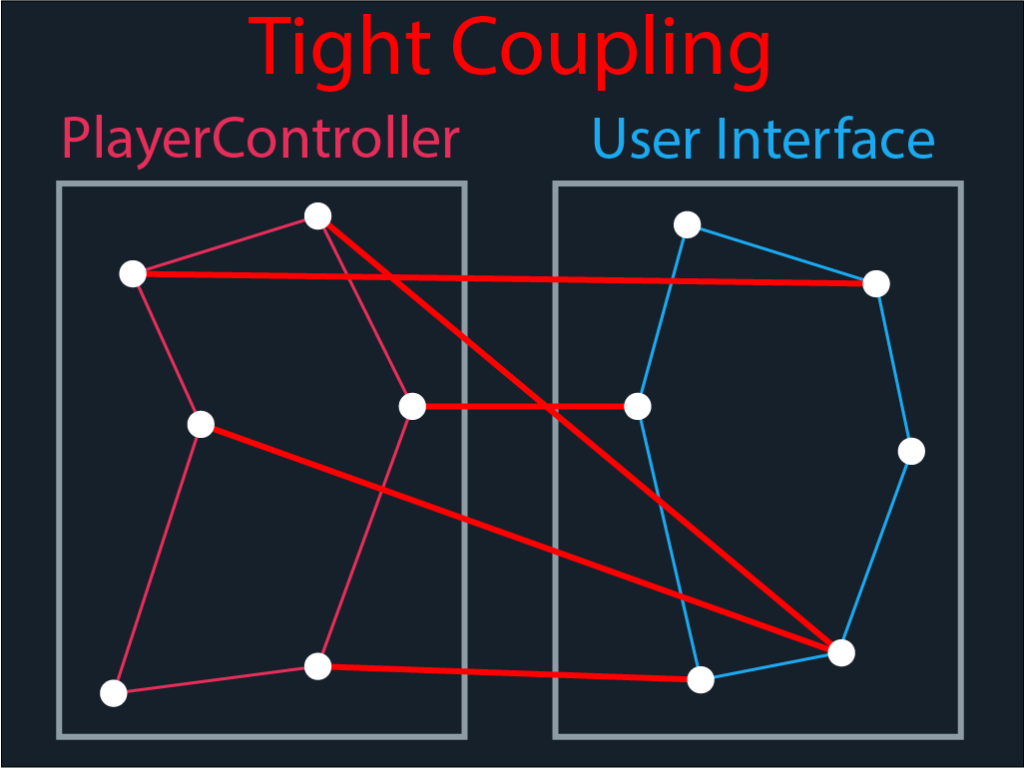
Loose coupling is generally preferred. Loose coupling means that two systems are minimally dependant on one another which in turn means that a change in one system might lead to none or at least only minimal changes in other systems.
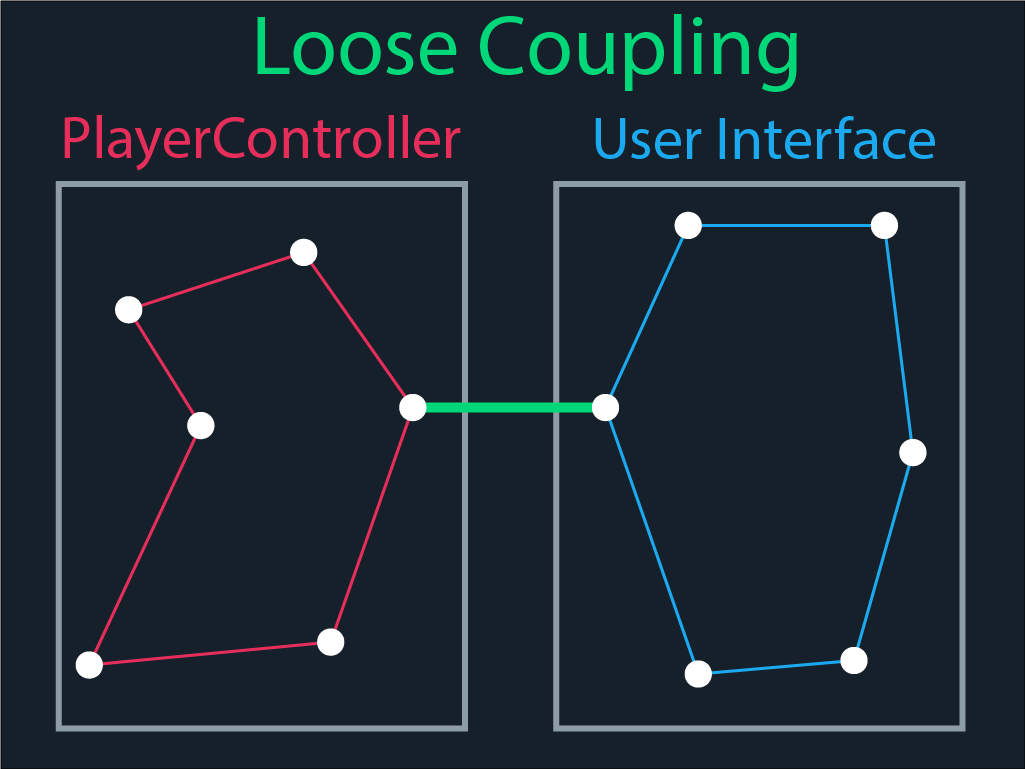
Practical Implications
What this means in practice is, that the PlayerController should usually never directly set i.e. the Score in the User-Interface. Instead it would be wiser to have a UI-Manager which handles the UI, its animations and so on.
Here is an example of what I mean:
class PlayerController : MonoBehaviour
{
[serializeField] private IngameUI _ui;
private int _hitpoints = 3;
void OnTriggerEnter(Collider col)
{
_hitpoints--;
_ui.UpdateHitpoints(_hitpoints);
}
}
class IngameUI : MonoBehaviour
{
[SerializeField] private HealthBar _healthBar;
[SerializeField] private PostProcessingManager _postProcessing;
private int _playerHp;
public void UpdateHitpoints(int newHp)
{
_playerHp = newHp;
ShakeScreen();
PlayUIAnimation(newHp);
_postProcessing.UpdatePostProcessingEffects();
_healthBar.Update(newHp);
}
//...
}
As you can see the IngameUI-Class is responsible for a bunch of things that need to happen when the player receives damage. The PlayerController now just tells the IngameUI about his new Health-Value and the IngameUI is responsible for reacting to this change by shaking the screen, telling the healthbar to update healthbar etc.
That way you can find bugs way more easily because if it has something to do with the UI you know for certain, that you don’t need to look into Player-Scripts.